SOLID Principles Interview Questions
The SOLID principles are fundamental to writing maintainable and scalable
software. Here are ten interview questions to test your understanding of these
principles.
1. What are the SOLID principles?
Answer: The SOLID principles are a set of five design principles aimed at
making software designs more understandable, flexible, and maintainable. They
are:
- Single Responsibility Principle (SRP)
- Open/Closed Principle (OCP)
- Liskov Substitution Principle (LSP)
- Interface Segregation Principle (ISP)
- Dependency Inversion Principle (DIP)
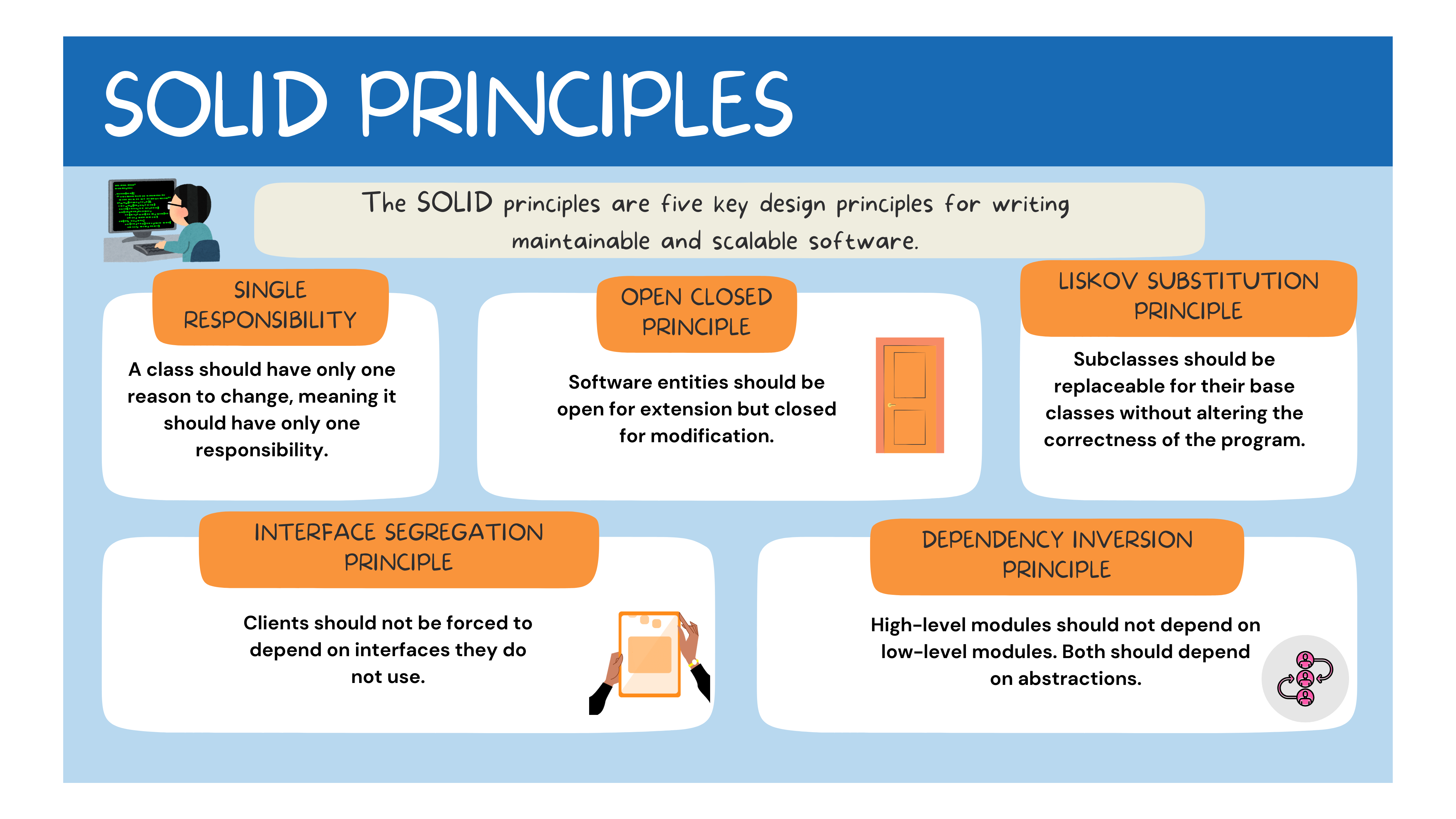
2. Can you explain the Single Responsibility Principle (SRP) with an example?
Answer: The Single Responsibility Principle states that a class should have
one, and only one, reason to change. This means that each class should have a
single responsibility or function.
Example:
class User {
private String name;
private String email;
public User(String name, String email) {
this.name = name;
this.email = email;
}
// Getters and setters...
}
class UserRepository {
public void save(User user) {
// Save user to database
}
}
class User {
constructor(name, email) {
this.name = name
this.email = email
}
}
class UserRepository {
save(user) {
}
}
class User:
def __init__(self, name, email):
self.name = name
self.email = email
class UserRepository:
def save(self, user):
3. What does the Open/Closed Principle (OCP) entail, and how can it be applied?
Answer: The Open/Closed Principle states that software entities (classes,
modules, functions, etc.) should be open for extension but closed for
modification. This means you should be able to add new functionality without
changing existing code.
Example:
abstract class Shape {
abstract double area();
}
class Circle extends Shape {
private double radius;
public Circle(double radius) {
this.radius = radius;
}
@Override
double area() {
return Math.PI * radius * radius;
}
}
class Rectangle extends Shape {
private double width;
private double height;
public Rectangle(double width, double height) {
this.width = width;
this.height = height;
}
@Override
double area() {
return width * height;
}
}
class Shape {
area() {
throw new Error('Method not implemented')
}
}
class Circle extends Shape {
constructor(radius) {
super()
this.radius = radius
}
area() {
return Math.PI * this.radius * this.radius
}
}
class Rectangle extends Shape {
constructor(width, height) {
super()
this.width = width
this.height = height
}
area() {
return this.width * this.height
}
}
class Shape:
def area(self):
raise NotImplementedError("Method not implemented")
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14159 * self.radius * self.radius
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * her.height
4. Describe the Liskov Substitution Principle (LSP) and provide an example.
Answer: The Liskov Substitution Principle states that objects of a
superclass should be replaceable with objects of a subclass without altering the
correctness of the program. This principle ensures that derived classes can be
used interchangeably with their base classes without causing errors.
Example:
class Bird {
public void fly() {
System.out.println("Flying");
}
}
class Duck extends Bird {
public void quack() {
System.out.println("Quacking");
}
}
class Penguin extends Bird {
@Override
public void fly() {
throw new UnsupportedOperationException("Penguins can't fly");
}
}
public class Main {
public static void main(String[] args) {
Bird duck = new Duck();
duck.fly(); // Works
Bird penguin = new Penguin();
penguin.fly(); // Throws exception
}
}
class Bird {
fly() {
console.log('Flying')
}
}
class Duck extends Bird {
quack() {
console.log('Quacking')
}
}
class Penguin extends Bird {
fly() {
throw new Error("Penguins can't fly")
}
}
function makeBirdFly(bird) {
bird.fly()
}
const duck = new Duck()
makeBirdFly(duck)
const penguin = new Penguin()
makeBirdFly(penguin)
class Bird:
def fly(self):
print("Flying")
class Duck(Bird):
def quack(self):
print("Quacking")
class Penguin(Bird):
def fly(self):
raise Exception("Penguins can't fly")
def make_bird_fly(bird):
bird.fly()
duck = Duck()
make_bird_fly(duck)
penguin = Penguin()
make_bird_fly(penguin)
5. What is the Interface Segregation Principle (ISP), and how does it improve software design?
Answer: The Interface Segregation Principle states that clients should not
be forced to depend on interfaces they do not use. This principle encourages the
creation of small, specific interfaces rather than large, general-purpose ones,
making your code more modular and easier to understand.
Example:
interface Printer {
void print();
}
interface Scanner {
void scan();
}
class MultiFunctionPrinter implements Printer, Scanner {
private Printer printer;
private Scanner scanner;
public MultiFunctionPrinter(Printer printer, Scanner scanner) {
this.printer = printer;
this.scanner = scanner;
}
@Override
public void print() {
printer.print();
}
@Override
public void scan() {
scanner.scan();
}
}
class Printer {
print() {
throw new Error('Method not implemented')
}
}
class Scanner {
scan() {
throw new Error('Method not implemented')
}
}
class MultiFunctionPrinter {
constructor(printer, scanner) {
this.printer = printer
this.scanner = scanner
}
print() {
this.printer.print()
}
scan() {
this.scanner.scan()
}
}
class Printer:
def print(self):
raise NotImplementedError("Method not implemented")
class Scanner:
def scan(self):
raise NotImplementedError("Method not implemented")
class MultiFunctionPrinter:
def __init__(self, printer, scanner):
self.printer = printer
self.scanner = scanner
def print(self):
self.printer.print()
def scan(self):
self.scanner.scan()
6. How does the Dependency Inversion Principle (DIP) help in creating flexible and maintainable software?
Answer: The Dependency Inversion Principle states that high-level modules
should not depend on low-level modules but both should depend on abstractions.
This principle reduces the coupling between different parts of your code, making
it more flexible and easier to maintain.
Example:
// Abstraction
interface PaymentMethod {
void process();
}
// High-Level Module
class PaymentProcessor {
private PaymentMethod payment;
public PaymentProcessor(PaymentMethod payment) {
this.payment = payment;
}
public void processPayment() {
payment.process();
}
}
// Low-Level Modules
class CreditCardPayment implements PaymentMethod {
@Override
public void process() {
System.out.println("Processing credit card payment");
}
}
class PayPalPayment implements PaymentMethod {
@Override
public void process() {
System.out.println("Processing PayPal payment");
}
}
// Usage
public class Main {
public static void main(String[] args) {
PaymentMethod paymentMethod = new CreditCardPayment();
PaymentProcessor processor = new PaymentProcessor(paymentMethod);
processor.processPayment(); // Outputs: Processing credit card payment
paymentMethod = new PayPalPayment();
processor = new PaymentProcessor(paymentMethod);
processor.processPayment(); // Outputs: Processing PayPal payment
}
}
class PaymentMethod {
process() {
throw new Error('This method should be overridden')
}
}
class PaymentProcessor {
constructor(payment) {
this.payment = payment
}
processPayment() {
this.payment.process()
}
}
class CreditCardPayment extends PaymentMethod {
process() {
console.log('Processing credit card payment')
}
}
class PayPalPayment extends PaymentMethod {
process() {
console.log('Processing PayPal payment')
}
}
const paymentMethod = new CreditCardPayment()
let processor = new PaymentProcessor(paymentMethod)
processor.processPayment()
const payPalPayment = new PayPalPayment()
processor = new PaymentProcessor(payPalPayment)
processor.processPayment()
from abc import ABC, abstractmethod
class PaymentMethod(ABC):
@abstractmethod
def process(self):
pass
class PaymentProcessor:
def __init__(self, payment):
self.payment = payment
def process_payment(self):
self.payment.process()
class CreditCardPayment(PaymentMethod):
def process(self):
print("Processing credit card payment")
class PayPalPayment(PaymentMethod):
def process(self):
print("Processing PayPal payment")
payment_method = CreditCardPayment()
processor = PaymentProcessor(payment_method)
processor.process_payment()
payment_method = PayPalPayment()
processor = PaymentProcessor(payment_method)
processor.process_payment()
7. How would you refactor a monolithic class that handles multiple responsibilities to adhere to the SRP?
Answer: To refactor a monolithic class that handles multiple
responsibilities, you should break down the class into smaller classes, each
handling a single responsibility. This involves identifying the different
responsibilities and creating separate classes for each.
Example:
// Monolithic class
class UserService {
public void createUser(String name, String email) {
// Create user
}
public void sendEmail(String email, String message) {
// Send email
}
}
// Refactored to adhere to SRP
class UserCreationService {
public void createUser(String name, String email) {
// Create user
}
}
class EmailService {
public void sendEmail(String email, String message) {
// Send email
}
}
class UserService {
createUser(name, email) {
}
sendEmail(email, message) {
}
}
class UserCreationService {
createUser(name, email) {
}
}
class EmailService {
sendEmail(email, message) {
}
}
class UserService:
def create_user(self, name, email):
pass
def send_email(self, email, message):
pass
class UserCreationService:
def create_user(self, name, email):
pass
class EmailService:
def send_email(self, email, message):
pass
8. Explain how you can apply the OCP to an existing class.
Answer: To apply the OCP to an existing class, you can use inheritance or
composition to add new functionality without modifying the existing class. This
involves creating new subclasses or components that extend or enhance the
original functionality.
Example:
// Existing class
class Notification {
public void send(String message) {
// Send notification
}
}
// Apply OCP using inheritance
class EmailNotification extends Notification {
@Override
public void send(String message) {
// Send email notification
}
}
class SMSNotification extends Notification {
@Override
public void send(String message) {
// Send SMS notification
}
}
class Notification {
send(message) {
}
}
class EmailNotification extends Notification {
send(message) {
}
}
class SMSNotification extends Notification {
send(message) {
}
}
class Notification:
def send(self, message):
pass
class EmailNotification(Notification):
def send(self, message):
pass
class SMSNotification(Notification):
def send(self, message):
pass
9. How does following the ISP improve code maintainability?
Answer: Following the Interface Segregation Principle (ISP) improves code
maintainability by creating small, specific interfaces rather than large,
general-purpose ones. This makes the code easier to understand, change, and
extend, as clients only depend on the interfaces they actually use.
Example:
interface Printer {
void print();
}
interface Scanner {
void scan();
}
class MultiFunctionPrinter implements Printer, Scanner {
private Printer printer;
private Scanner scanner;
public MultiFunctionPrinter(Printer printer, Scanner scanner) {
this.printer = printer;
this.scanner = scanner;
}
@Override
public void print() {
printer.print();
}
@Override
public void scan() {
scanner.scan();
}
}
class Printer {
print() {
throw new Error('Method not implemented')
}
}
class Scanner {
scan() {
throw new Error('Method not implemented')
}
}
class MultiFunctionPrinter {
constructor(printer, scanner) {
this.printer = printer
this.scanner = scanner
}
print() {
this.printer.print()
}
scan() {
this.scanner.scan()
}
}
class Printer:
def print(self):
raise NotImplementedError("Method not implemented")
class Scanner:
def scan(self):
raise NotImplementedError("Method not implemented")
class MultiFunctionPrinter:
def __init__(self, printer, scanner):
self.printer = printer
self.scanner = scanner
def print(self):
self.printer.print()
def scan(self):
self.scanner.scan()
10. How can the DIP help in testing your code?
Answer: The Dependency Inversion Principle (DIP) helps in testing your code
by allowing you to inject dependencies, making it easier to replace real
implementations with mocks or stubs during testing. This improves testability
and enables you to write more effective unit tests.
Example:
// Abstraction
interface PaymentMethod {
void process();
}
// High-Level Module
class PaymentProcessor {
private PaymentMethod payment;
public PaymentProcessor(PaymentMethod payment) {
this.payment = payment;
}
public void processPayment() {
payment.process();
}
}
// Mock for testing
class MockPaymentMethod implements PaymentMethod {
@Override
public void process() {
System.out.println("Mock payment processed");
}
}
// Test
public class PaymentProcessorTest {
public static void main(String[] args) {
PaymentMethod mockPayment = new MockPaymentMethod();
PaymentProcessor processor = new PaymentProcessor(mockPayment);
processor.processPayment(); // Outputs: Mock payment processed
}
}
class PaymentMethod {
process() {
throw new Error('This method should be overridden')
}
}
class PaymentProcessor {
constructor(payment) {
this.payment = payment
}
processPayment() {
this.payment.process()
}
}
class MockPaymentMethod extends PaymentMethod {
process() {
console.log('Mock payment processed')
}
}
const mockPayment = new MockPaymentMethod()
const processor = new PaymentProcessor(mockPayment)
processor.processPayment()
from abc import ABC, abstractmethod
class PaymentMethod(ABC):
@abstractmethod
def process(self):
pass
class PaymentProcessor:
def __init__(self, payment):
self.payment = payment
def process_payment(self):
self.payment.process()
class MockPaymentMethod(PaymentMethod):
def process(self):
print("Mock payment processed")
mock_payment = MockPaymentMethod()
processor = PaymentProcessor(mock_payment)
processor.process_payment()
11. How does the Single Responsibility Principle (SRP) help in reducing code complexity?
Answer: The Single Responsibility Principle (SRP) helps in reducing code
complexity by ensuring that each class or module has only one responsibility or
reason to change. This makes the code more modular and easier to understand,
test, and maintain. By separating concerns, you can isolate changes and minimize
the impact on other parts of the codebase.
12. What are some common violations of the Open/Closed Principle (OCP)?
Answer: Common violations of the Open/Closed Principle include:
- Modifying existing classes to add new functionality instead of extending them.
- Using conditional statements (like if-else or switch) to handle new types or
behaviors, rather than using polymorphism.
- Not using abstractions (interfaces or abstract classes) to define new
behavior.
13. How can you ensure that a class adheres to the Liskov Substitution Principle (LSP)?
Answer: To ensure a class adheres to the Liskov Substitution Principle, you
should:
- Ensure that derived classes can be used interchangeably with their base
classes without altering the expected behavior.
- Avoid overriding methods in derived classes in a way that violates the
contract established by the base class.
- Use design by contract, where preconditions cannot be strengthened and
postconditions cannot be weakened in the derived class compared to the base
class.
14. Can you provide an example of how the Interface Segregation Principle (ISP) improves system design?
Answer: The Interface Segregation Principle (ISP) improves system design by
creating small, specific interfaces rather than large, general-purpose ones.
This makes the system more modular and easier to maintain. For example:
interface Printer {
void print();
}
interface Scanner {
void scan();
}
class MultiFunctionPrinter implements Printer, Scanner {
private Printer printer;
private Scanner scanner;
public MultiFunctionPrinter(Printer printer, Scanner scanner) {
this.printer = printer;
this.scanner = scanner;
}
@Override
public void print() {
printer.print();
}
@Override
public void scan() {
scanner.scan();
}
}
class Printer {
print() {
throw new Error('Method not implemented')
}
}
class Scanner {
scan() {
throw new Error('Method not implemented')
}
}
class MultiFunctionPrinter {
constructor(printer, scanner) {
this.printer = printer
this.scanner = scanner
}
print() {
this.printer.print()
}
scan() {
this.scanner.scan()
}
}
class Printer:
def print(self):
raise NotImplementedError("Method not implemented")
class Scanner:
def scan(self):
raise NotImplementedError("Method not implemented")
class MultiFunctionPrinter:
def __init__(self, printer, scanner):
self.printer = printer
self.scanner = scanner
def print(self):
self.printer.print()
def scan(self):
self.scanner.scan()
15. Describe a scenario where not following the Dependency Inversion Principle (DIP) could lead to problems.
Answer: Not following the Dependency Inversion Principle can lead to tightly
coupled code, making it difficult to change or extend. For example, if a
high-level module directly depends on a low-level module (without using
abstractions), any change in the low-level module could require changes in the
high-level module, increasing the risk of bugs and making the code harder to
maintain.
16. How does the Open/Closed Principle (OCP) support the addition of new features?
Answer: The Open/Closed Principle supports the addition of new features by
allowing classes to be extended without modifying existing code. This is
typically achieved through inheritance or composition. By relying on
abstractions, new functionality can be added by creating new classes that extend
the existing ones, thus preserving the integrity of the original codebase.
17. Can you explain the importance of the Liskov Substitution Principle (LSP) with a real-world analogy?
Answer: The Liskov Substitution Principle (LSP) can be understood with a
real-world analogy of a car rental service. Imagine you rent a car, and you
expect it to drive like any other car you've driven. If you replace the car with
a truck, it should still operate like a car (with a steering wheel, pedals,
etc.) and fulfill the basic expectations. If the truck requires you to use
different controls, it would violate LSP, as it wouldn't meet the expected
behavior of a car.
18. How can the Interface Segregation Principle (ISP) prevent changes in one part of a system from affecting other parts?
Answer: The Interface Segregation Principle (ISP) prevents changes in one
part of a system from affecting other parts by ensuring that clients depend only
on the interfaces they use. This means that changes to one interface do not
impact other interfaces. For example, if a printer interface is modified, it
won't affect scanner functionality if they are segregated into separate
interfaces.
19. What are the benefits of using Dependency Injection to adhere to the Dependency Inversion Principle (DIP)?
Answer: Using Dependency Injection to adhere to the Dependency Inversion
Principle provides several benefits:
- Decoupling: It reduces the dependency between high-level and low-level
modules.
- Flexibility: It allows for easy swapping of implementations without
changing the code.
- Testability: It makes the code easier to test by allowing mock
implementations to be injected during testing.
- Maintainability: It simplifies the code by centralizing the creation and
management of dependencies.
20. How does applying SOLID principles collectively improve the overall design of a software system?
Answer: Applying SOLID principles collectively improves the overall design
of a software system by:
- Enhancing modularity and separation of concerns.
- Making the system more adaptable to change and extension.
- Improving readability and maintainability.
- Increasing testability and reducing the risk of bugs.
- Promoting code reuse and reducing redundancy.
21. What are the common signs that indicate a violation of the Single Responsibility Principle (SRP)?
Answer: Common signs of SRP violations include:
- A class has multiple responsibilities or reasons to change.
- Methods within a class are highly interdependent, leading to complex and
tangled code.
- Difficulty in understanding, testing, or maintaining a class because it does
too many things.
22. How does the Open/Closed Principle (OCP) enhance software maintenance?
Answer: The Open/Closed Principle enhances software maintenance by allowing
developers to add new features or functionalities without altering existing
code. This minimizes the risk of introducing bugs into stable code, ensures
backward compatibility, and makes the system easier to extend over time.
23. Can you describe the relationship between the Liskov Substitution Principle (LSP) and polymorphism?
Answer: The Liskov Substitution Principle (LSP) is closely related to
polymorphism, as both concepts involve using a base class or interface to
interact with objects. LSP ensures that subclasses or derived classes can be
substituted for their base class without altering the expected behavior, making
polymorphism effective and reliable in software design.
24. What are the advantages of applying the Interface Segregation Principle (ISP) in large systems?
Answer: Applying ISP in large systems offers several advantages:
- Reduced Complexity: By breaking down large interfaces into smaller,
specific ones, the system becomes easier to understand and maintain.
- Improved Flexibility: Changes in one part of the system are less likely to
impact other parts, enhancing modularity.
- Better Testing: Smaller interfaces are easier to test in isolation.
- Enhanced Collaboration: Different teams can work on different parts of the
system without interfering with each other's work.
25. How can dependency injection frameworks help in adhering to the Dependency Inversion Principle (DIP)?
Answer: Dependency injection frameworks help in adhering to DIP by:
- Managing the creation and injection of dependencies automatically.
- Decoupling high-level modules from low-level modules, as dependencies are
injected at runtime.
- Simplifying the configuration and management of dependencies, making the
system more flexible and easier to maintain.
26. Provide an example of how violating the Single Responsibility Principle (SRP) can lead to maintenance issues.
Answer: Violating SRP can lead to maintenance issues such as:
- Example: A
UserService
class that handles user creation, validation,
authentication, and email notifications. - Issues: Any change in the user creation logic might affect other
functionalities like authentication or email notifications, making the class
difficult to maintain and test.
27. How can the Open/Closed Principle (OCP) be implemented using interfaces and abstract classes?
Answer: OCP can be implemented using interfaces and abstract classes by
defining an abstraction for the behavior that can be extended. Concrete
implementations can then be created to extend this behavior without modifying
the existing code.
Example:
abstract class Notification {
abstract void send(String message);
}
class EmailNotification extends Notification {
@Override
void send(String message) {
System.out.println("Sending email: " + message);
}
}
class SMSNotification extends Notification {
@Override
void send(String message) {
System.out.println("Sending SMS: " + message);
}
}
class Notification {
send(message) {
throw new Error('Method not implemented')
}
}
class EmailNotification extends Notification {
send(message) {
console.log('Sending email: ' + message)
}
}
class SMSNotification extends Notification {
send(message) {
console.log('Sending SMS: ' + message)
}
}
from abc import ABC, abstractmethod
class Notification(ABC):
@abstractmethod
def send(self, message):
pass
class EmailNotification(Notification):
def send(self, message):
print('Sending email:', message)
class SMSNotification(Notification):
def send(self, message):
print('Sending SMS:', message)
28. Why is it important for subclasses to adhere to the Liskov Substitution Principle (LSP)?
Answer: It is important for subclasses to adhere to LSP to ensure that the
system remains reliable and predictable. Adhering to LSP means that subclasses
can be used interchangeably with their base classes without causing unexpected
behaviors or errors, thus maintaining the integrity and correctness of the
program.
29. How can you apply the Interface Segregation Principle (ISP) to a monolithic interface?
Answer: To apply ISP to a monolithic interface, you can refactor the
interface into multiple smaller, more specific interfaces that each serve a
distinct purpose. Clients can then implement only the interfaces they need,
reducing unnecessary dependencies.
Example:
// Monolithic interface
interface Worker {
void work();
void eat();
}
// Refactored interfaces
interface Workable {
void work();
}
interface Eatable {
void eat();
}
class WorkerImpl implements Workable, Eatable {
@Override
public void work() {
System.out.println("Working");
}
@Override
public void eat() {
System.out.println("Eating");
}
}
class Worker {
work() {
throw new Error('Method not implemented')
}
eat() {
throw new Error('Method not implemented')
}
}
class Workable {
work() {
throw new Error('Method not implemented')
}
}
class Eatable {
eat() {
throw new Error('Method not implemented')
}
}
class WorkerImpl extends Workable {
work() {
console.log('Working')
}
eat() {
console.log('Eating')
}
}
from abc import ABC, abstractmethod
class Worker(ABC):
@abstractmethod
def work(self):
pass
@abstractmethod
def eat(self):
pass
class Workable(ABC):
@abstractmethod
def work(self):
pass
class Eatable(ABC):
@abstractmethod
def eat(self):
pass
class WorkerImpl(Workable, Eatable):
def work(self):
print('Working')
def eat(self):
print('Eating')
30. What is the role of abstraction in the Dependency Inversion Principle (DIP)?
Answer: Abstraction plays a crucial role in DIP by decoupling high-level
modules from low-level modules. By depending on abstractions rather than
concrete implementations, high-level modules can remain unaffected by changes in
low-level modules, resulting in a more flexible and maintainable system.
Abstractions, such as interfaces or abstract classes, allow for different
implementations to be swapped easily without altering the high-level module.
31. How does adhering to the Single Responsibility Principle (SRP) improve testing?
Answer: Adhering to the Single Responsibility Principle improves testing by
making each class or module responsible for only one functionality. This
isolation makes it easier to write unit tests, as each test can focus on a
single behavior. It also simplifies the creation of mock objects and reduces the
likelihood of side effects during testing.
32. What is the impact of violating the Open/Closed Principle (OCP) on system extensibility?
Answer: Violating the Open/Closed Principle impacts system extensibility by
requiring modifications to existing code when new functionality is added. This
increases the risk of introducing bugs and reduces the ability to extend the
system smoothly. Adhering to OCP allows new features to be added through
extensions rather than changes, preserving the stability of the existing
codebase.
33. Can you explain the relationship between the Liskov Substitution Principle (LSP) and interface contracts?
Answer: The Liskov Substitution Principle is closely related to interface
contracts, as it requires that subclasses honor the contracts defined by their
base classes. This means that subclasses must fulfill the expected behavior and
constraints of the interfaces they implement. Violating these contracts can lead
to unexpected behavior and errors when using subclasses interchangeably with
their base classes.
34. How can you identify if a class is violating the Interface Segregation Principle (ISP)?
Answer: A class is likely violating the Interface Segregation Principle if
it implements methods from an interface that it does not use. This can be
identified by:
- Checking for methods that are left unimplemented or throw exceptions.
- Analyzing the class to see if it depends on methods it doesn't actually need.
- Identifying large, monolithic interfaces that serve multiple clients with
different requirements.
35. How does the Dependency Inversion Principle (DIP) facilitate easier refactoring?
Answer: The Dependency Inversion Principle facilitates easier refactoring by
decoupling high-level modules from low-level modules. By relying on
abstractions, changes in low-level modules do not directly affect high-level
modules. This allows for easier updates, replacements, and improvements to
individual components without impacting the overall system, simplifying the
refactoring process.
36. What are some practical benefits of following the Single Responsibility Principle (SRP)?
Answer: Practical benefits of following SRP include:
- Easier Maintenance: Isolated responsibilities make it simpler to locate
and fix bugs.
- Improved Readability: Code is more understandable and easier to navigate.
- Enhanced Reusability: Classes with single responsibilities are more likely
to be reusable in different contexts.
- Simpler Testing: Each class can be tested independently, leading to more
robust unit tests.
37. How does the Open/Closed Principle (OCP) help in achieving code reusability?
Answer: The Open/Closed Principle helps in achieving code reusability by
allowing existing code to be extended rather than modified. This encourages the
creation of reusable components that can be combined or extended to add new
functionality, promoting the reuse of stable and tested code across different
parts of the application.
38. Can you describe a scenario where the Liskov Substitution Principle (LSP) is crucial for API design?
Answer: In API design, LSP is crucial when designing an inheritance
hierarchy for client usage. For example, if an API provides a base class
Animal
with a method makeSound()
, any subclass like Dog
or Cat
must
implement makeSound()
in a way that conforms to the expected behavior.
Violating LSP by having a subclass that throws exceptions or behaves differently
can break client code that relies on the API, leading to a poor user experience.
39. How does the Interface Segregation Principle (ISP) contribute to a modular system architecture?
Answer: The Interface Segregation Principle contributes to a modular system
architecture by promoting the use of small, specific interfaces. This allows
different parts of the system to depend only on the interfaces they need,
reducing unnecessary dependencies and making the system more modular. Each
module can be developed, tested, and maintained independently, leading to a more
flexible and scalable architecture.
40. What are the challenges of implementing the Dependency Inversion Principle (DIP) in legacy systems?
Answer: Challenges of implementing DIP in legacy systems include:
- High Coupling: Legacy systems often have tightly coupled components,
making it difficult to introduce abstractions.
- Refactoring Complexity: Introducing abstractions and dependency injection
can require significant refactoring, which may be risky without comprehensive
tests.
- Resistance to Change: Team members may resist changes due to the perceived
complexity and potential disruptions to existing functionality.
- Lack of Tests: Legacy systems may lack sufficient tests, making it harder
to ensure that refactoring does not introduce new bugs.
41. How can the Single Responsibility Principle (SRP) help in managing technical debt?
Answer: The Single Responsibility Principle helps in managing technical debt
by ensuring that each class or module has a single responsibility. This reduces
the complexity of the code, making it easier to understand, maintain, and
refactor. By isolating changes to specific parts of the code, SRP minimizes the
risk of introducing new bugs, thus preventing the accumulation of technical
debt.
42. What is the difference between the Open/Closed Principle (OCP) and the Single Responsibility Principle (SRP)?
Answer: The Single Responsibility Principle (SRP) focuses on ensuring that a
class or module has only one reason to change, emphasizing separation of
concerns. The Open/Closed Principle (OCP) emphasizes that software entities
should be open for extension but closed for modification, allowing new
functionality to be added without altering existing code. While SRP ensures
focused responsibilities, OCP ensures extensibility without modifying stable
code.
43. How does the Liskov Substitution Principle (LSP) enhance code reusability?
Answer: The Liskov Substitution Principle enhances code reusability by
ensuring that subclasses can be used interchangeably with their base classes
without altering the correctness of the program. This allows developers to
create reusable components and extend existing classes without breaking the
functionality of the application, promoting the reuse of tested and reliable
code.
44. Can you provide an example where violating the Interface Segregation Principle (ISP) leads to bloated classes?
Answer: Violating the Interface Segregation Principle can lead to bloated
classes when a single interface contains methods that are not relevant to all
implementing classes. For example:
// Violating ISP
interface Worker {
void work();
void eat();
}
class RobotWorker implements Worker {
@Override
public void work() {
System.out.println("Working");
}
@Override
public void eat() {
throw new UnsupportedOperationException("Robots don't eat");
}
}
class Worker {
work() {
throw new Error('Method not implemented')
}
eat() {
throw new Error('Method not implemented')
}
}
class RobotWorker extends Worker {
work() {
console.log('Working')
}
eat() {
throw new Error("Robots don't eat")
}
}
class Worker:
def work(self):
raise NotImplementedError("Method not implemented")
def eat(self):
raise NotImplementedError("Method not implemented")
class RobotWorker(Worker):
def work(self):
print("Working")
def eat(self):
raise Exception("Robots don't eat")
45. How can the Dependency Inversion Principle (DIP) improve the scalability of an application?
Answer: The Dependency Inversion Principle improves the scalability of an
application by decoupling high-level modules from low-level modules. This allows
for easier integration of new features and technologies without modifying
existing code. By relying on abstractions, the application can be extended and
scaled by adding new implementations, thus maintaining a flexible and modular
architecture.
46. What are the key differences between the Interface Segregation Principle (ISP) and the Single Responsibility Principle (SRP)?
Answer: The Interface Segregation Principle (ISP) focuses on creating small,
specific interfaces that clients depend on, ensuring that they only interact
with the methods they use. The Single Responsibility Principle (SRP) focuses on
ensuring that a class or module has only one reason to change, emphasizing
separation of concerns. While ISP deals with interface design and client
interactions, SRP deals with class design and responsibility allocation.
47. How does the Open/Closed Principle (OCP) facilitate better collaboration among developers?
Answer: The Open/Closed Principle facilitates better collaboration among
developers by allowing new functionality to be added through extensions rather
than modifications. This means that multiple developers can work on extending
different parts of the system simultaneously without interfering with each
other's work. It also reduces the risk of conflicts and bugs, as existing code
remains unchanged and stable.
48. Can you explain how the Liskov Substitution Principle (LSP) can prevent runtime errors?
Answer: The Liskov Substitution Principle prevents runtime errors by
ensuring that subclasses can be used interchangeably with their base classes
without altering the expected behavior. This adherence to the base class
contract ensures that client code works correctly with any subclass instance,
reducing the risk of unexpected behavior and runtime errors caused by incorrect
subclass implementations.
49. How does the Interface Segregation Principle (ISP) support the principle of least privilege?
Answer: The Interface Segregation Principle supports the principle of least
privilege by ensuring that clients depend only on the interfaces they actually
need. This minimizes the exposure of unnecessary methods and reduces the risk of
clients inadvertently using methods they shouldn't. By providing specific
interfaces tailored to client requirements, ISP ensures that clients have access
only to the functionalities they require, enhancing security and
maintainability.
50. What are some common challenges when applying the Dependency Inversion Principle (DIP) in practice?
Answer: Common challenges when applying the Dependency Inversion Principle
include:
- Identifying Abstractions: Determining the appropriate abstractions can be
difficult, especially in complex systems.
- Refactoring Legacy Code: Introducing abstractions and dependency injection
into existing code can require significant refactoring and testing.
- Performance Considerations: Using abstractions and dependency injection
frameworks can introduce performance overhead.
- Complexity: The added complexity of managing dependencies through
abstractions and injection can be a hurdle for developers not familiar with
these concepts.
Additional Resources
Books:
- Clean Code: A Handbook of Agile Software Craftsmanship by Robert C. Martin
- Clean Architecture: A Craftsman's Guide to Software Structure and Design
by Robert C. Martin
Articles:
Youtube Links: